What Page object mode?
-Page object model in simple term is an design pattern you create in your Test Framework which helps you to manage your code.
lets look at the example below,
POM is design patter we will create to manage our code easily.
in which we will create a Two classes.
One class is for defining all the locators and actions of a page.
Second class is for using that locators and actions and perform operation on it.
Why We should choose POM?
Non POM layout:-
In Non POM layout means without any design pattern suppose we are writing a Login test than what we do in it is
Step 1 :-
Create a java class than we define WebDriver and than Define WebElement and using that WebElement we perform action on it in that class.
like,
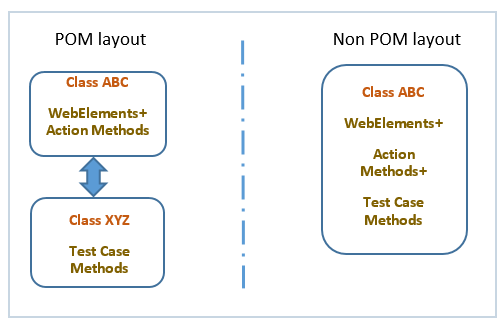
POM is design patter we will create to manage our code easily.
in which we will create a Two classes.
One class is for defining all the locators and actions of a page.
Second class is for using that locators and actions and perform operation on it.
Non POM layout:-
In Non POM layout means without any design pattern suppose we are writing a Login test than what we do in it is
Step 1 :-
Create a java class than we define WebDriver and than Define WebElement and using that WebElement we perform action on it in that class.
like,
package facebook; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.firefox.FirefoxDriver; public class facebook2 { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver","C:\\Users\\5558\\eclipse-workspace\\chromedriver.exe"); System.out.println("hello"); WebDriver driver = new ChromeDriver(); System.out.println("hello"); driver.get("https://www.facebook.com"); System.out.println("hello"); driver.manage().window().maximize(); driver.findElement(By.xpath(".//*[@id='email']")).sendKeys("divyangjani@gmail.com"); driver.findElement(By.xpath(".//*[@id='pass']")).sendKeys("dixit1234"); driver.findElement(By.xpath(".//*[@id='u_0_2']")).click(); driver.quit(); } }
Suppose , after creating this because of some reason Locator of any button is changed. so in this case we have to go to the every place in our code and change that element's locator manually.
and this action will be very time consuming and annoying.
But,
and this action will be very time consuming and annoying.
But,
If we Use design pattern of POM than our code will be :-
This is the First class in which we are defining all the locators of a page.
package facebook; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; public class webelement { static WebDriver driver; static WebElement element; public webelement(WebDriver driver) { //super(); this.driver = driver; } public static WebElement firstname() { element=driver.findElement(By.xpath("//input[@name='firstname']")); return element; } public static WebElement lastname() { element=driver.findElement(By.xpath("//input[@name='lastname']")); return element; } public static WebElement email() { element=driver.findElement(By.xpath("//input[@name='reg_email__']")); return element; } public static WebElement newpassword() { element=driver.findElement(By.xpath("//input[@name='reg_passwd__']")); return element; } public static WebElement day() { element=driver.findElement(By.xpath("//select[@name='birthday_day']")); return element; } public static WebElement month() { element=driver.findElement(By.xpath("//select[@name='birthday_month']")); return element; } public static WebElement year() { element=driver.findElement(By.xpath("//select[@name='birthday_year']")); return element; } public static WebElement sex() { element=driver.findElement(By.xpath("(//input[@name='sex'])[2]")); return element; } public static WebElement signup() { element=driver.findElement(By.xpath("//button[@name='websubmit']")); return element; } public void set_firstname(String fname) { firstname().sendKeys(fname); } }
And this is the class where we will use this locators and actions to perform operation.
package facebook; import java.io.File; import java.io.IOException; import java.util.concurrent.TimeUnit; import org.openqa.selenium.OutputType; import org.openqa.selenium.TakesScreenshot; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.io.FileHandler; import org.openqa.selenium.support.ui.Select; import org.testng.annotations.AfterTest; import org.testng.annotations.Test; import facebook.facebook; import wixlogin.TakeScreenshot; public class signup { static WebDriver driver; @Test public void login() { System.setProperty("webdriver.chrome.driver","C:\\Users\\5558\\eclipse-workspace\\chromedriver.exe"); driver=new ChromeDriver(); driver.get("https://www.facebook.com"); driver.manage().timeouts().implicitlyWait(2000, TimeUnit.MILLISECONDS); driver.manage().window().maximize(); webelement ele = new webelement(driver); //ele.firstname().sendKeys("dixit"); ele.set_firstname("test"); ele.lastname().sendKeys("jani"); ele.email().sendKeys("test@gmail.com"); ele.newpassword().sendKeys("dixit1234"); Select day = new Select(ele.day()); day.selectByIndex(24); Select month = new Select(ele.month()); month.selectByVisibleText("Jul"); Select year = new Select(webelement.year()); year.selectByVisibleText("1997"); ele.sex().click(); ele.signup().click(); } @AfterTest public void close_browser() { driver.close(); } }
Now if you look at this code and Non POM class code , you will notice that it is very eye catching to easy and easy to handle. while Non POM code looks complex to understand what is going on?
I hope this article helps you :)
if you like this article than please do share it with your freinds/colleagues.
No comments:
Post a Comment